Short Story Featuring SpecialOne
SpecialOne Demo
Project Description
Now that we’re sold on the idea of buying SpecialOne, let’s first learn its basics.
Who am I?
I’m the founder of SpecialOne. SpecialOne is a surprise box expert that elevates your engagement day by curating your glowing and music ring box.
Why is my company relevant to you?
In today’s ultra-competitive world, the only way people can feel good about themselves is by being better than others. It is so easy for people to get lost in the comparison loop. It’s common for people to have second thoughts after seeing their ring and go about thinking – “Jess’s ring looks better than mine! Ugh! Why did John even propose me with this single solitaire ring?” However, this ruins the entire essence of a special moment that can only be experienced by you and no one else.
SpecialOne aims at enhancing the engagement day experience by removing unnecessary pressure on how fancy your ring should be, but instead works on making the moment special.
Who would buy SpecialOne Surprise Box?
The market for SpecialOne Surprise Boxes is huge! According to wedding.report (yes, this website exists!) the total number of weddings in 2020 was 1.2 million in the United States. If we get a 10% of this market share, then we would get roughly 120,000 buyers in the US alone!
Who will mentor me in this project?
I would seek assistance from the course instructor, Professor St. John and Instructor Mensing.
What materials and funding will I require for this project?
I would require:
- Squarewear
- Arduino UNO
- Wooden Ply
- Drilling Equipment
- Screws
- Saw
- Red fancy cloth
- Willpower
Documenting My Progress
Day 2
I learned how to solder and it was fun! Soldering did take a ton of time, but finally seeing this neopixel band light up was extremely satisfying.
Day 3
Day 4
Component Connections
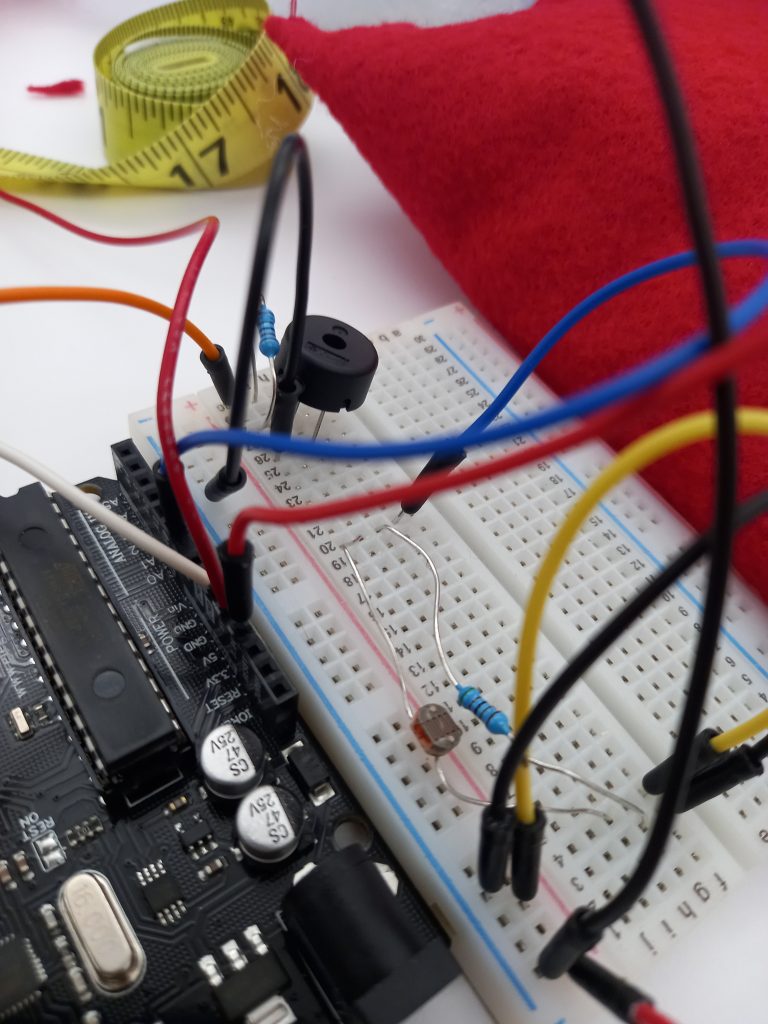
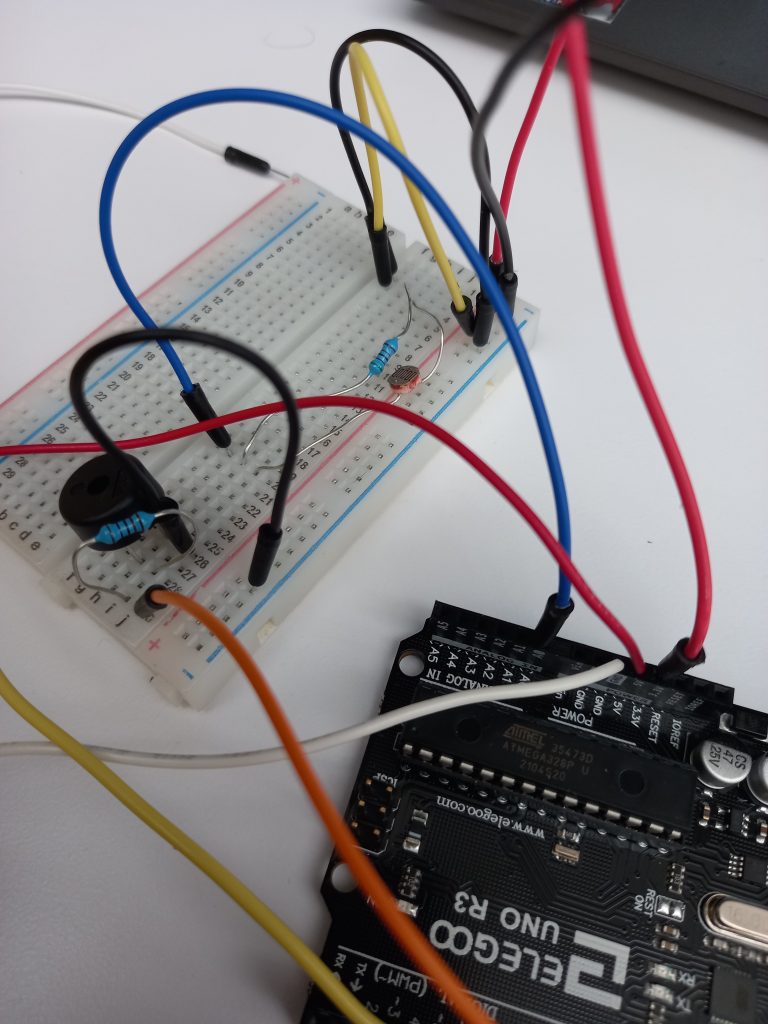
My Code
// NeoPixel Ring simple sketch (c) 2013 Shae Erisson
// Released under the GPLv3 license to match the rest of the
// Adafruit NeoPixel library
#include <Adafruit_NeoPixel.h>
#ifdef __AVR__
#include <avr/power.h> // Required for 16 MHz Adafruit Trinket
#endif
//-----------ALL CONSTANTS AND PIN CONNECTIONS --------------------------
// Which pin on the Arduino is connected to the NeoPixels?
#define PIN 6 // Neopixel Attachment
#define BUZZER_PIN 9 //Where is the buzzer attached
const int ldrPin = A0;
#define DELAYVAL 500 // Time (in milliseconds) to pause between pixels
#define NUMPIXELS 16 // Popular NeoPixel ring size // How many NeoPixels are attached to the Arduino?
#define LED_COUNT 12
// When setting up the NeoPixel library, we tell it how many pixels,
// and which pin to use to send signals. Note that for older NeoPixel
// strips you might need to change the third parameter -- see the
// strandtest example for more information on possible values.
Adafruit_NeoPixel pixels(NUMPIXELS, PIN, NEO_GRB + NEO_KHZ800);
Adafruit_NeoPixel strip(LED_COUNT, PIN, NEO_GRB + NEO_KHZ800);
void setup() {
Serial.begin(9600);
pinMode(PIN, OUTPUT);
pinMode(ldrPin, INPUT);
pixels.begin(); // INITIALIZE NeoPixel strip object (REQUIRED)
pixels.clear();
}
void loop() {
int ldrStatus = analogRead(ldrPin);
//CHECKINGG FOR LIGHT
if (ldrStatus <= 200)
{
Serial.print("Its Dark, Turn on the LED:");
Serial.println(ldrStatus);
pixels.clear(); // Set all pixel colors to 'off'
}
else
{
Serial.print("Its Bright, Turn off the LED:");
Serial.println(ldrStatus);
pixels.clear(); // Set all pixel colors to 'off'
//Now turn the light on
pixels.show(); // Send the updated pixel colors to the hardware.
// The first NeoPixel in a strand is #0, second is 1, all the way up
// to the count of pixels minus one.
for(int i=0; i<NUMPIXELS; i++) { // For each pixel...
// pixels.Color() takes RGB values, from 0,0,0 up to 255,255,255
theaterChase(strip.Color(150, 0, 0), 50); // Red, half brightness
playSong();
theaterChase(strip.Color(127, 127, 127), 50); // White, half brightness
theaterChase(strip.Color(127, 0, 0), 50); // Red, half brightness
theaterChase(strip.Color( 0, 0, 127), 50); // Blue, half brightness
rainbow(100); // Flowing rainbow cycle along the whole strip
}
}
}
// Add the notes needed using the note sheet. Spaces acts like breaks
char song[] = "mmggaag mmggaag mmeeddc mmffeed mmggaag mmggadm";
// beats per note
// Add the beats needed to complete the song.
// The amount of beats should match the amount of notes and spaces
int beats[] = { 5, 1, 1, 7, 1, 3, 2, 4,
5, 1, 1, 7, 1, 3, 2, 4,
1, 3, 4, 2, 2, 1, 1, 2,
5, 1, 1, 7, 1, 3, 2, 4,
5, 1, 1, 7, 1, 3, 2, 4,
5, 1, 1, 7, 1, 3, 2, 4};
//------------Modify ONLY The Code Above To Update the Melody ----------
//---------------- Do Not Edit The Code Below------------------------
// speed of song
int tempo = 300;
// convenience notes means we don't need Pitches.h
// names of the notes
char names[] = { 'c', 'd', 'e', 'f', 'g', 'a', 'b', 'C' };
// corresponding tones for C4 - B4, C5 (looked up values from Pitches.h)
int tones[] = { 262, 295, 330, 349, 392, 440, 494, 523 };
// play the song using the variables: song, songLength, beats
void playSong()
{
delay( 5000 );
// for each note in the melody:
for (int noteIndex = 0; noteIndex < sizeof(song); noteIndex++)
{
// if it's a space
if (song[noteIndex] == ' ')
{
// rest
delay( beats[noteIndex] * tempo/5);
}
// otherwise it's the name of a note
else
{
// play the note at that index for the specified time
playNote(song[noteIndex], beats[noteIndex] * tempo);
}
// pause between notes
delay(tempo);
}
}
// play the tone corresponding to the note name
void playNote(char noteName, int duration)
{
// loop through the names
for (int i = 0; i < sizeof(names); i++)
{
// if we found the right name
if (names[i] == noteName)
{
// play the tone at the same index
tone( BUZZER_PIN, tones[i], duration);
// don't bother looking through the rest of the names
break;
}
}
}
//------------------Additional Neopixel functions----------------------------
void theaterChase(uint32_t color, int wait) {
for(int a=0; a<10; a++) { // Repeat 10 times...
for(int b=0; b<3; b++) { // 'b' counts from 0 to 2...
strip.clear(); // Set all pixels in RAM to 0 (off)
// 'c' counts up from 'b' to end of strip in steps of 3...
for(int c=b; c<strip.numPixels(); c += 3) {
strip.setPixelColor(c, color); // Set pixel 'c' to value 'color'
}
strip.show(); // Update strip with new contents
delay(wait); // Pause for a moment
}
}
}
// Rainbow cycle along whole strip. Pass delay time (in ms) between frames.
void rainbow(int wait) {
// Hue of first pixel runs 5 complete loops through the color wheel.
// Color wheel has a range of 65536 but it's OK if we roll over, so
// just count from 0 to 5*65536. Adding 256 to firstPixelHue each time
// means we'll make 5*65536/256 = 1280 passes through this loop:
for(long firstPixelHue = 0; firstPixelHue < 5*65536; firstPixelHue += 256) {
// strip.rainbow() can take a single argument (first pixel hue) or
// optionally a few extras: number of rainbow repetitions (default 1),
// saturation and value (brightness) (both 0-255, similar to the
// ColorHSV() function, default 255), and a true/false flag for whether
// to apply gamma correction to provide 'truer' colors (default true).
strip.rainbow(firstPixelHue);
// Above line is equivalent to:
// strip.rainbow(firstPixelHue, 1, 255, 255, true);
strip.show(); // Update strip with new contents
delay(wait); // Pause for a moment
}
}