Scientists define self-regulated learning as a cyclic process of setting tasks, developing strategies, evaluating the effectiveness of those strategies, and making necessary changes to reach one’s goal.
However, most of us as college students believe self-regulated learning seems easier said than done. It takes constant time (which college students lack) and energy to be implemented on a regular basis. Under the mountain of responsibilities, constant decision-making, adjustments, and assignments, it is difficult for one to pause and truly reflect on their learning strategy.
I followed the same school of thought until recently when I had to take the time out to complete this assignment. When I sat down to reflect on what I learned from the assignment, I realized that jumping haphazardly into a project might not be the best way of accomplishing it.
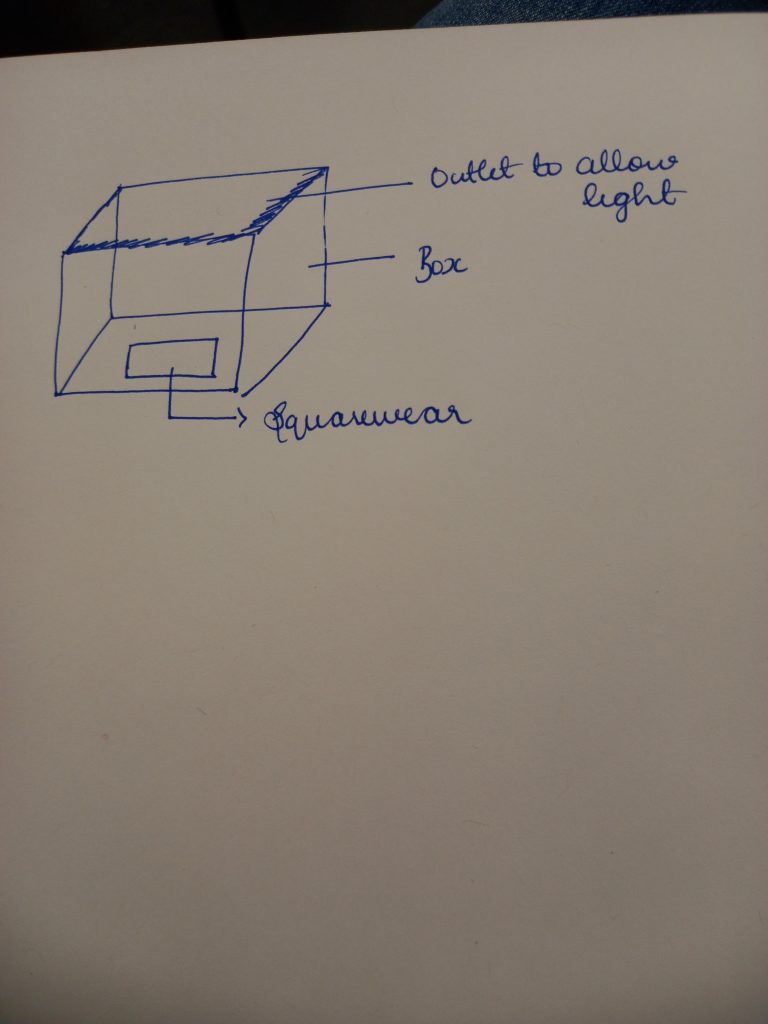
I followed the same school of thought until recently when I had to take the time out to complete this assignment. When I sat down to reflect on what I learned from the assignment, I realized that jumping haphazardly into a project might not be the best way of accomplishing it. You may then ask the following question:
What is the biggest hindrance to self-regulated learning?
We’re always on the go. When we are constantly surrounded by people who are always on the go and never seem to stop, we tend to fall into the same trap. It appears as if it is always necessary to be working on something, doing something, becoming someone. This constant pressure of doing something deprives us of the time where we can actually sit down and reflect on our learning journey so far.
It appears as if we live to follow a to-do list and not the other way around. That is why it is important to first accept that we should not be filling every single minute of our day with “productivity”. That is often the opposite of productivity.
How Did I Learn To Self Regulate Through This Project?
I realized that I should always come up with a plan in order to complete something successfully. More often than not, I jump into a project haphazardly, only to realize that I have wasted my precious time and energy that could have been spent doing other important things.
Next, I learned that most problems are simpler than we make them appear. If a project isn’t working even after we spent countless hours working on it, we must learn to either ask for help or let it go. Considering it as the end of the world would not help in magically fixing the project.
Finally, I learned that there are concepts and ideas that occur to us with time. A part of self-regulated learning is to acknowledge that we will not immediately find answers to everything, but at least we would have the knowledge of recognizing what is working and what is not.
Code
/*
Melody
Plays a melody on SquareWear
* use buzzer on digital pin 9
Written by Shani Mensing, edited by Audrey St. John
From example code in the public domain.
http://arduino.cc/en/Tutorial/Tone
*/
// Output for SquareWear
// specific to SquareWear
#define BUZZER_PIN 9
// squarewear specific
#define LIGHT_SENSOR_PIN A0
// current index of note that is playing
int currentNoteIndex = 0;
// deciding if there's enough light
// increase to require more ambient light before the led is on
// decrease to require less
int minLightValue = 100;
// notes for the song. A space represents a rest
char song[]= "ccggaag ffeeddc ggffeed ggffeed ccggaag ffeeddc ";
// the number of notes in the song
int songLength = 30;
// beats per note
int beats[] = { 1, 1, 1, 1, 1, 1, 2, 1, 1, 1, 1, 1, 1, 2,
1, 1, 2, 1, 1, 1, 2, 2, 1, 1, 1, 1, 1, 1, 4};
// speed of song
int tempo = 300;
// names of the notes
char names[] = { 'c', 'd', 'e', 'f', 'g', 'a', 'b', 'C', 'D', 'E', 'F', 'G' };
// corresponding tones for C4 - B4, C5-G5 from Pitches.h
int tones[] = { 262, 295, 330, 349, 392, 440, 494, 523, 587, 659, 698, 784 };
// number of notes
int numberConvenienceNotes = 12;
void setup()
{
Serial.begin(9600);
if(checkBrightness())
( BUZZER_PIN, OUTPUT );
}
boolean checkBrightness()
{
int lightValue = analogRead(LIGHT_SENSOR_PIN);
if (lightValue >= minLightValue)
return true;
else
return false;
}
// play the next note in the song using the variables: song, songLength, beats
void playNextNote()
{
// if it's a space
if (song[currentNoteIndex] == ' ')
{
// rest
delay( beats[currentNoteIndex] * tempo/5);
}
// otherwise it's the name of a note
else
{
// play the note at that index for the specified time
playNote(song[currentNoteIndex], beats[currentNoteIndex] * tempo);
}
// pause between notes
delay(tempo);
currentNoteIndex = (currentNoteIndex+1)%songLength;
}
// play the tone corresponding to the note name
void playNote(char noteName, int duration)
{
if(checkBrightness()){
// loop through the names
for (int i = 0; i < numberConvenienceNotes; i++)
{
// if we found the right name
if (names[i] == noteName)
{
// play the tone at the same index
tone( BUZZER_PIN, tones[i], duration);
// don't bother looking through the rest of the names
break;
}
}
}
}